- Published on
Eddie Bowles AR Tour - 2023 Update
Walking Tour - Fast forward to 2023
Eddie's walking tour has been in the app stores for about a year now. As of January 2023 we have 62 installed devices! See my previous blog post for some more background on this project.
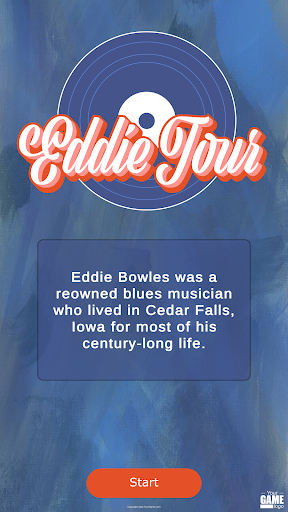
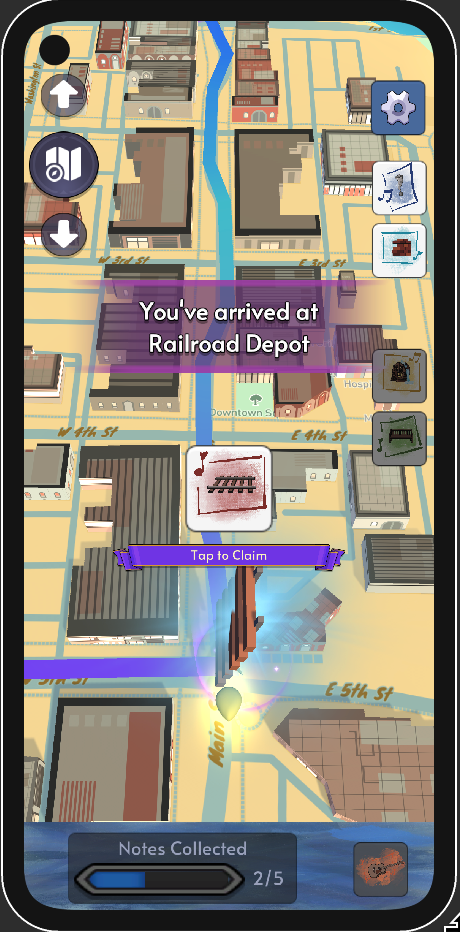
Eddie and his tour have a few locations on the web -
Eddie has also been covered in "Living Blues Review".
Most importantly, Eddie's music has been made publicly available on Spotify, CD, Amazon, and many other platforms.
Code Odds and Ends
RectTransformExtensions
I found this gist quite handy for my waypoint UI. Using that, we can simply use the following two lines of code...
waypoint.button.GetComponent<RectTransform>().SetAnchor(AnchorPresets.MiddleCenter, 0, -10f, ease: Ease.InOutQuart);
waypoint.button.transform.DOScale(1.6f, 1.5f).SetEase(Ease.InOutQuart).Play();
...to provide this animation.
TourLineSpawner
Pretty simple LineRenderer given a set of points. This is how I render the tour line. Mapbox provides a nice utility to convert between latlongs and scene world Vector3
s.
public class TourLineSpawner : MonoBehaviour
{
[Geocode] public List<string> locations;
public AbstractMap map;
public LineRenderer lineRenderer;
public int offsetY;
private void Update()
{
lineRenderer.SetPositions(locations.Select(l =>
{
var geo = map.GeoToWorldPosition(Conversions.StringToLatLon(l), true);
return new Vector3(geo.x, geo.y + offsetY, geo.z);
}
).ToArray());
}
}
Waypoints
The waypoints work about how you'd expect too. They are prefabs that are instantiated when the scene is loaded based on a little list of structs.
[Serializable]
public struct EddieWaypoint
{
[AssetSelector] public Sprite icon2d;
[AssetSelector] public GameObject icon3d;
public float icon3dScale;
[AssetSelector] public GameObject detailModal;
public string name;
[Geocode] public string location;
}
The user's location has a trigger collider. When it enters the bounds of one of the waypoints, that's when we know to highlight the waypoint to learn more about it.
public class PlayerTarget : MonoBehaviour
{
public GameState gameState;
private void OnTriggerEnter(Collider other)
{
var el = other.gameObject.GetComponent<EddieLocation>();
if (el != null && el.PositionWasSet())
{
gameState.OnEnterEddieLocation(el);
}
}
private void OnTriggerExit(Collider other)
{
var el = other.gameObject.GetComponent<EddieLocation>();
if (el != null && el.PositionWasSet())
{
gameState.OnLeaveEddieLocation();
}
}
}
GameState is very simple for for this app. We implement those two previous OnEnter/OnLeave functions as well as a function to mark waypoints as visited.
public class GameState : MonoBehaviour
{
public LocationText locationText;
public HashSet<EddieWaypoint> LocationsFound { get; } = new();
public ButtonSpawner buttonSpawner;
public EddieWaypoint[] waypoints;
public EddieLocation activeLocation;
public bool IsZoomFull { get; set; }
public void OnEnterEddieLocation(EddieLocation el)
{
if (LocationsFound.Contains(el.eddieWaypoint))
{
return;
}
activeLocation = el;
locationText.EnterWaypoint(el.eddieWaypoint);
buttonSpawner.EnterWaypoint(el.eddieWaypoint);
}
public void OnLeaveEddieLocation()
{
if (!activeLocation) return;
locationText.LeaveWaypoint();
buttonSpawner.LeaveWaypoint(activeLocation!.eddieWaypoint);
activeLocation = null;
}
public void OnFind(EddieWaypoint eddieLocation)
{
LocationsFound.Add(eddieLocation);
OnLeaveEddieLocation();
}
}
Walking Tour Ideas
I've considered spinning this idea out into a SaaS product. I think it'd be a fun product to create your own walking tours!
Consider -
A unique anniversary present for your significant other. Choose locations in your town that are relevant to your relationship and make it a fun challenge with a surprise at the end! Even better, a scavenger hunt with puzzles to unlock the next waypoint.
A celebration of life. For folks coming in from out of town, it would add more meaning to the event if you didn't know the person very well. And something to bond over with the other attendees.
Tourism. A draw and reason to visit a location. For example, a driving tour around Hawaii's Big Island or a tour around food & drink establishments in Wisconsin's Door County.
Maybe someday in the next life when I have more free time :smile: